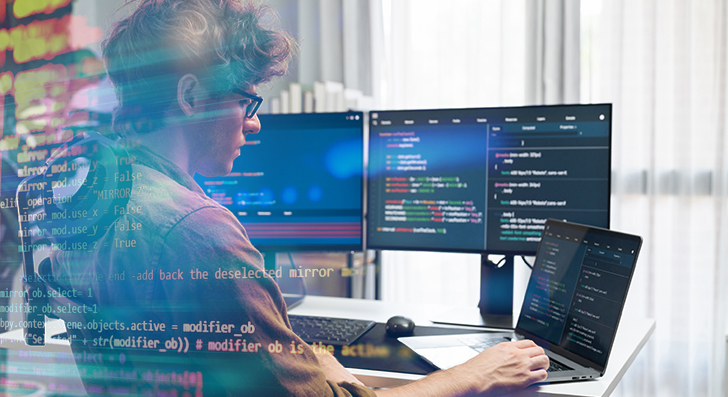
Scalability suggests your application can handle advancement—far more consumers, much more details, plus more targeted traffic—with no breaking. As being a developer, setting up with scalability in mind will save time and tension afterwards. Right here’s a transparent and functional manual to help you start out by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is just not one thing you bolt on afterwards—it should be section of the plan from the beginning. Many programs fail every time they expand speedy since the first style can’t cope with the extra load. Being a developer, you should Imagine early about how your process will behave under pressure.
Commence by building your architecture to become versatile. Avoid monolithic codebases in which every thing is tightly linked. In its place, use modular style or microservices. These designs crack your application into smaller, impartial sections. Each module or assistance can scale By itself with out impacting The full procedure.
Also, think about your database from day one particular. Will it have to have to deal with 1,000,000 people or simply just a hundred? Choose the correct variety—relational or NoSQL—based upon how your details will grow. Prepare for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
Another essential level is in order to avoid hardcoding assumptions. Don’t publish code that only performs beneath existing problems. Give thought to what would materialize if your user foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use design and style designs that help scaling, like concept queues or function-driven techniques. These aid your app manage a lot more requests without having having overloaded.
When you build with scalability in your mind, you are not just making ready for fulfillment—you might be reducing long term headaches. A nicely-planned procedure is less complicated to keep up, adapt, and develop. It’s much better to prepare early than to rebuild afterwards.
Use the appropriate Database
Picking out the proper database is often a critical Portion of creating scalable programs. Not all databases are constructed the same, and utilizing the Mistaken you can slow you down or simply bring about failures as your app grows.
Get started by comprehension your info. Can it be hugely structured, like rows inside a desk? If Of course, a relational database like PostgreSQL or MySQL is a superb in shape. They are potent with associations, transactions, and regularity. Additionally they aid scaling tactics like read replicas, indexing, and partitioning to take care of far more visitors and facts.
In case your facts is more adaptable—like user action logs, products catalogs, or paperwork—think about a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured knowledge and will scale horizontally a lot more conveniently.
Also, think about your examine and write styles. Are you undertaking many reads with fewer writes? Use caching and browse replicas. Will you be handling a hefty publish load? Take a look at databases that may tackle higher compose throughput, or maybe party-based info storage programs like Apache Kafka (for non permanent data streams).
It’s also intelligent to Feel forward. You might not need Superior scaling characteristics now, but picking a databases that supports them suggests you received’t need to switch later.
Use indexing to speed up queries. Steer clear of unneeded joins. Normalize or denormalize your facts based upon your obtain styles. And normally observe databases performance when you mature.
To put it briefly, the right database depends upon your app’s structure, velocity requires, And exactly how you hope it to improve. Acquire time to choose properly—it’ll conserve a great deal of difficulties later on.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, every single tiny delay provides up. Inadequately prepared code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s vital that you Develop efficient logic from the beginning.
Start off by creating clean, very simple code. Prevent repeating logic and remove something pointless. Don’t pick the most advanced Remedy if a simple just one performs. Keep your capabilities quick, focused, and simple to test. Use profiling applications to uncover bottlenecks—spots exactly where your code usually takes also long to operate or makes use of too much memory.
Following, take a look at your databases queries. These frequently gradual factors down more than the code by itself. Make sure Just about every query only asks for the information you truly want. Stay clear of Pick *, which fetches all the things, and alternatively find precise fields. Use indexes to speed up lookups. And keep away from accomplishing too many joins, In particular throughout huge tables.
For those who discover precisely the same data getting asked for many times, use caching. Keep the results temporarily employing applications like Redis or Memcached so that you don’t must repeat high priced functions.
Also, batch your database operations once you can. In place of updating a row one after the other, update them in teams. This cuts down on overhead and can make your application extra efficient.
Remember to check with massive datasets. Code and queries that get the job done great with 100 records may crash whenever they have to manage one million.
In short, scalable apps are quick apps. Keep your code tight, your queries lean, and use caching when required. These measures support your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's got to take care of additional buyers plus more traffic. If everything goes through one server, it will quickly turn into a bottleneck. That’s the place load balancing and caching can be found in. These two resources support maintain your app quick, stable, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As an alternative to one particular server carrying out each of the function, the load balancer routes users to distinctive servers based upon availability. This suggests no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other individuals. Resources like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this simple to setup.
Caching is about storing details briefly so it can be reused immediately. When end users request exactly the same information and facts yet again—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. You may serve it with the cache.
There are two popular sorts of caching:
1. Server-aspect caching (like Redis or Memcached) shops details in memory for quickly obtain.
2. Shopper-side caching (like browser caching or CDN caching) outlets static information near the user.
Caching lowers databases load, enhances velocity, and can make your app a lot more economical.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when data does modify.
To put it briefly, load balancing and caching are straightforward but highly effective tools. Collectively, they assist your app cope with more consumers, continue to be fast, and Recuperate from challenges. If you propose to develop, you may need both of those.
Use Cloud and Container Resources
To create scalable apps, you would like tools that allow your app develop simply. That’s wherever cloud platforms and containers are available. They give you flexibility, minimize setup time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and providers as you may need them. You don’t should obtain hardware or read more guess long term capability. When site visitors will increase, it is possible to insert additional methods with just some clicks or mechanically applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and stability tools. You can focus on setting up your application in place of controlling infrastructure.
Containers are One more crucial Instrument. A container packages your application and almost everything it should run—code, libraries, configurations—into a person device. This makes it easy to maneuver your application amongst environments, out of your laptop into the cloud, devoid of surprises. Docker is the most well-liked tool for this.
Once your app utilizes various containers, instruments like Kubernetes enable you to handle them. Kubernetes handles deployment, scaling, and Restoration. If a single component within your app crashes, it restarts it automatically.
Containers also make it very easy to independent aspects of your app into services. You may update or scale elements independently, which happens to be great for performance and dependability.
In brief, working with cloud and container resources usually means you'll be able to scale speedy, deploy simply, and Get better swiftly when problems transpire. If you'd like your application to grow devoid of limits, start off applying these resources early. They help save time, reduce chance, and help you remain centered on setting up, not fixing.
Keep an eye on All the things
Should you don’t watch your software, you won’t know when points go wrong. Monitoring will help the thing is how your application is performing, place troubles early, and make improved decisions as your app grows. It’s a crucial Component of setting up scalable methods.
Commence by tracking primary metrics like CPU use, memory, disk House, and reaction time. These tell you how your servers and solutions are undertaking. Applications like Prometheus, Grafana, Datadog, or New Relic can help you gather and visualize this info.
Don’t just keep an eye on your servers—keep an eye on your application far too. Regulate how much time it's going to take for buyers to load internet pages, how frequently faults materialize, and where they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital complications. As an example, Should your response time goes above a Restrict or simply a company goes down, it is best to get notified promptly. This will help you correct concerns quickly, frequently before buyers even see.
Checking is additionally helpful when you make variations. When you deploy a whole new characteristic and see a spike in glitches or slowdowns, it is possible to roll it back again prior to it causes authentic hurt.
As your app grows, targeted visitors and knowledge improve. Without checking, you’ll skip indications of problems until it’s far too late. But with the correct tools in position, you stay on top of things.
In short, checking assists you keep the app trusted and scalable. It’s not nearly recognizing failures—it’s about comprehending your method and making certain it works properly, even under pressure.
Ultimate Views
Scalability isn’t just for significant organizations. Even compact apps will need a strong Basis. By building very carefully, optimizing sensibly, and using the appropriate applications, you'll be able to Establish apps that increase effortlessly without having breaking stressed. Start tiny, Assume large, and Establish intelligent.